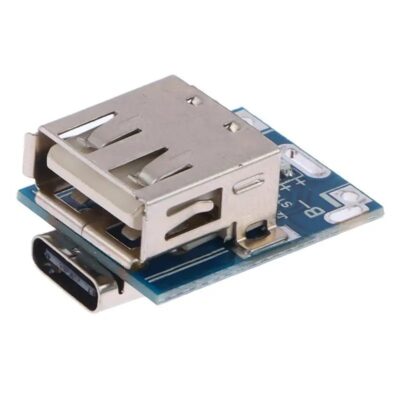
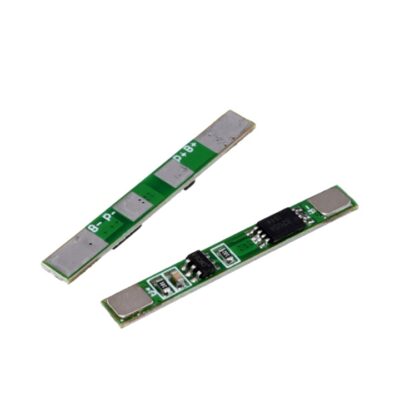
سنسور دما و رطوبت DHT22 AM2302
۲۵۳,۰۰۰ تومان
موجود در انبار
DHT22 AM2302 is a digital sensor that allows to obtain temperature and relative humidity readings, it is low cost and excellent performance, it is integrated by a capacitive humidity sensor and a thermistor to measure the surrounding air, which displays the data through a digital signal on the data pin.
It is used in automatic temperature control applications, air conditioning, environmental monitoring in agriculture, weather stations, home applications, humidity and temperature regulator.
SPECIFICATIONS AND FEATURES
Model: DHT22 AM2302
Operating voltage: 3.3 V to 5.5 V
Current Consumption: 2.5mA
Output signal: Digital
Digital interface: Standard digital single-bus (bidirectional)
Sampling rate: 2 seconds
Resolution: 16 bit
Measuring range Temperature: -40°C to 125°C
Temperature measurement accuracy: <±0.5 °C
Temperature Resolution: 0.1°C
Measuring range Humidity: 0% to 100% RH 0.5°C variation
Humidity measurement accuracy: 2% RH
Resolution Humidity: 0.1% RH
Maximum data cable distance: 20 m
Dimensions: 20mm x 15mm x 8mm
Example
The DHT11 and DHT22 sensors use their own bidirectional communication system through a single conductor, using timed signals.
In each measurement sending, the sensor sends a total of 40 bits, in 4 ms.
These 40 bits correspond to 2 Bytes for humidity measurement, 2 Bytes for temperature measurement, plus a final Byte for error checking (8bit integral RH data + 8bit decimal RH data + 8bit integral T data + 8bit decimal T data + 8bit checksum)
We can read the sensor data directly by generating and reading the timed signals according to the DHTXX protocol.
In general, it is normal for us to use an existing library to simplify the process.
There are several libraries available.
For example, we can use the Adafruit library available at this link.
We download and install the library and load the example code, or the following simplified version
#include "DHT.h"
// Uncomment whatever type you're using!
//#define DHTTYPE DHT11 // DHT 11
#define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321
//#define DHTTYPE DHT21 // DHT 21 (AM2301)
// Connect pin 1 (on the left) of the sensor to +5V
// NOTE: If using a board with 3.3V logic like an Arduino Due connect pin 1
// to 3.3V instead of 5V!
// Connect pin 2 of the sensor to whatever your DHTPIN is
// Connect pin 4 (on the right) of the sensor to GROUND
// Connect a 10K resistor from pin 2 (data) to pin 1 (power) of the sensor
const int DHTPin = 5; // what digital pin we're connected to
DHT dht(DHTPin, DHTTYPE);
void setup() {
Serial.begin(9600);
Serial.println("DHTxx test!");
dht.begin();
}
void loop() {
// Wait a few seconds between measurements.
delay(2000);
// Reading temperature or humidity takes about 250 milliseconds!
float h = dht.readHumidity();
float t = dht.readTemperature();
if (isnan(h) || isnan(t)) {
Serial.println("Failed to read from DHT sensor!");
return;
}
Serial.print("Humidity: ");
Serial.print(h);
Serial.print(" %\t");
Serial.print("Temperature: ");
Serial.print(t);
Serial.print(" *C ");
}
We load the program into Arduino, and we will begin to receive the temperature and humidity values through the serial port.
Pins
VCC: Pin (+) Connection to power supply from 3.3V to 5.5V
DATA: Outputs temperature and humidity through serial data
NC: No connection, do not use
GND: Pin (-) Connection to GND or Ground of the power supply
DHT22 is a digital sensor focused on measuring temperature and relative humidity.
It is a low-cost sensor with good performance, it will help you create different temperature and humidity control and monitoring projects.
How to connect the DHT22?
It is only necessary to connect the power VCC pin to 3.3V or 5V, the GND pin to Ground (0V), connect a resistor in Pull-up mode, between the Data pin – VCC and the data pin connect to a digital pin.
it is compatiblewith Arduino / Raspberry Pi / Nodemcu / ESP32 boards or any microcontroller that has digital pins.
It requires a 4.7K or 10k Ohm resistor in Pull-up mode, between the Data pin and VCC.
The following image defines how to connect the DHT22: